2-Legged OAuth is a useful authorization mechanism for apps that need to manipulate calendars on behalf of users in an organization. Both developers building apps for the
Google Apps Marketplace and domain administrators writing tools for their own domains can benefit. Let’s take a look at how to do this with the new
Calendar API v3 and
Java client library 1.6.0 beta.
To get started as a domain administrator, you need to explicitly enable the new Google Calendar API scopes under
Google Apps cPanel > Advanced tools > Manage your OAuth access > Manage third party OAuth Client access:
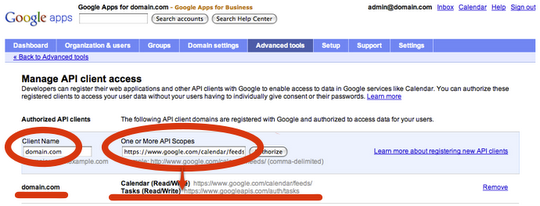
The scope to include is:
https://www.googleapis.com/auth/calendar
To do the same for a Marketplace app, include the scope in your
application's manifest.
Calendar API v3 also needs an API access key that can be retrieved in the
APIs Console. Once these requirements are taken care of, a new Calendar service object can be initialized:
public Calendar buildService() {
HttpTransport transport = AndroidHttp.newCompatibleTransport();
JacksonFactory jsonFactory = new JacksonFactory();
// The 2-LO authorization section
OAuthHmacSigner signer = new OAuthHmacSigner();
signer.clientSharedSecret = "";
final OAuthParameters oauthParameters = new OAuthParameters();
oauthParameters.version = "1";
oauthParameters.consumerKey = "";
oauthParameters.signer = signer;
Calendar service = Calendar.builder(transport, jsonFactory)
.setApplicationName("")
.setJsonHttpRequestInitializer(new JsonHttpRequestInitializer() {
@Override
public void initialize(JsonHttpRequest request) {
CalendarRequest calendarRequest = (CalendarRequest) request;
calendarRequest.setKey("");
}
}).setHttpRequestInitializer(oauthParameters).build();
return service;
}
Once the Calendar service object is properly initialized, it can be used to send authorized requests to the API. To access calendar data for a particular user, set the query parameter
xoauth_requestor_id
to a user’s email address:
public void printEvents() {
Calendar service = buildService();
// Add the xoauth_requestor_id query parameter to let the API know
// on behalf of which user the request is being made.
ArrayMap customKeys = new ArrayMap();
customKeys.add("xoauth_requestor_id", "");
List listEventsOperation = service.events().list("primary");
listEventsOperation.setUnknownKeys(customKeys);
Events events = listEventsOperation.execute();
for (Event event : events.getItems()) {
System.out.println("Event: " + event.getSummary());
}
}
Additionally, if the same service will be used to send requests on behalf of the same user, the
xoauth_requestor_id
query parameter can be set in the initializer:
// …
Calendar service = Calendar.builder(transport, jsonFactory)
.setApplicationName("")
.setJsonHttpRequestInitializer(new JsonHttpRequestInitializer() {
@Override
public void initialize(JsonHttpRequest request) {
ArrayMap customKeys = new ArrayMap();
customKeys.add("xoauth_requestor_id", "");
calendarRequest.setKey("");
calendarRequest.setUnknownKeys(customKeys);
}
}).setHttpRequestInitializer(oauthParameters).build();
// ...
We hope you’ll try out 2-Legged OAuth and let us know what you think in the
Google Calendar API forum.
No comments:
Post a Comment